In computer science, a Set is a data structure that stores a collection of unique elements. It is typically implemented using a hash table or a binary search tree, and provides efficient operations for adding, removing, and checking for the presence of elements. Sets are commonly used in algorithms and data processing tasks where duplicate elements are not allowed or need to be removed. They are also used in database systems to enforce uniqueness constraints on data. Keep reading below to learn how to use a Set in Javascript.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
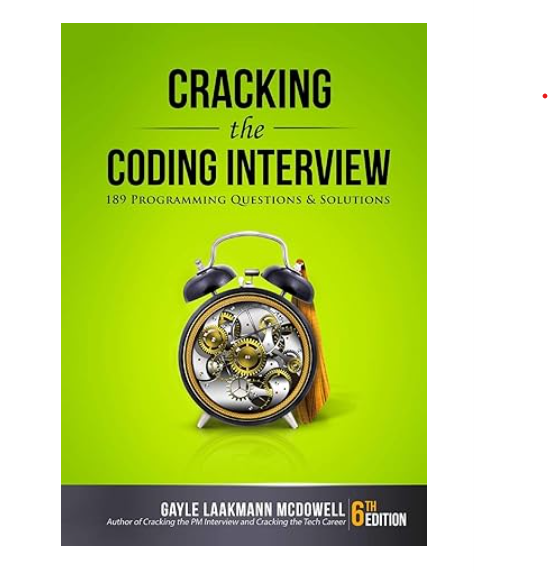
How to use a Set in Javascript with example code
A Set is a built-in object in JavaScript that allows you to store unique values of any type. In this blog post, we will explore how to use a Set in JavaScript with example code.
To create a new Set, you can use the following syntax:
const mySet = new Set();
This will create an empty Set. You can also pass an iterable object to the Set constructor to initialize it with values:
const mySet = new Set([1, 2, 3]);
To add values to a Set, you can use the add()
method:
mySet.add(4);
This will add the value 4 to the Set. If you try to add a value that already exists in the Set, it will be ignored.
To check if a value exists in a Set, you can use the has()
method:
mySet.has(4); // true
This will return true if the value exists in the Set, and false otherwise.
To remove a value from a Set, you can use the delete()
method:
mySet.delete(4);
This will remove the value 4 from the Set.
You can also get the size of a Set using the size
property:
mySet.size; // 3
This will return the number of values in the Set.
One of the main benefits of using a Set is that it automatically removes duplicates. For example:
const mySet = new Set([1, 2, 2, 3, 3, 3]);
This will create a Set with the values 1, 2, and 3. The duplicates are automatically removed.
In conclusion, Sets are a powerful tool in JavaScript for storing unique values of any type. They are easy to use and provide built-in methods for adding, removing, and checking values.
What is a Set in Javascript?
In conclusion, a set in JavaScript is a collection of unique values that can be easily manipulated and accessed using built-in methods. Sets are useful for removing duplicates from arrays, checking for the presence of a value, and performing mathematical operations such as union, intersection, and difference. With the introduction of sets in ES6, JavaScript developers now have a powerful tool at their disposal for working with collections of data. Whether you’re building a web application or working on a data analysis project, understanding how to use sets in JavaScript can help you write more efficient and effective code.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |