In computer science, a Set is a data structure that stores a collection of unique elements. It is typically implemented using a hash table or a binary search tree, and provides efficient operations for adding, removing, and checking for the presence of elements. Sets are commonly used in algorithms and data processing tasks where duplicate elements are not allowed or need to be removed. They are also used in database systems to enforce uniqueness constraints on data. Keep reading below to learn how to use a Set in PHP.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
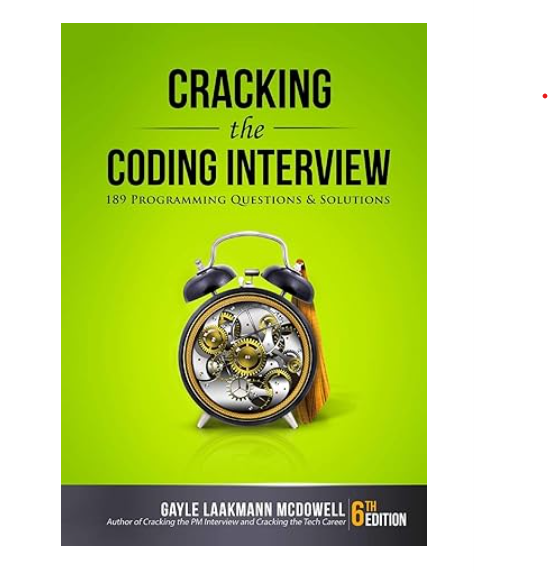
How to use a Set in PHP with example code
A Set is a collection of unique values in PHP. It is similar to an array, but it does not allow duplicate values. In this blog post, we will discuss how to use a Set in PHP with example code.
To use a Set in PHP, we need to first create an instance of the Set class. We can do this using the following code:
$set = new Set();
Once we have created a Set instance, we can add values to it using the add() method. For example, to add the values “apple”, “banana”, and “orange” to the Set, we can use the following code:
$set->add("apple");
$set->add("banana");
$set->add("orange");
To check if a value exists in the Set, we can use the has() method. For example, to check if the value “apple” exists in the Set, we can use the following code:
$set->has("apple"); // returns true
To remove a value from the Set, we can use the delete() method. For example, to remove the value “banana” from the Set, we can use the following code:
$set->delete("banana");
We can also get the size of the Set using the size() method. For example, to get the size of the Set, we can use the following code:
$set->size(); // returns 2
In conclusion, a Set is a useful data structure in PHP for storing unique values. We can create a Set instance using the Set class, add values to it using the add() method, check if a value exists in the Set using the has() method, remove a value from the Set using the delete() method, and get the size of the Set using the size() method.
What is a Set in PHP?
In conclusion, a set in PHP is a collection of unique values that can be manipulated using various set operations such as union, intersection, and difference. It is a useful data structure that allows developers to efficiently manage and manipulate data in their PHP applications. With the built-in set functions and methods available in PHP, developers can easily create, modify, and manipulate sets to suit their specific needs. Whether you are working on a small project or a large-scale application, understanding the concept of sets in PHP can help you write more efficient and effective code. So, if you haven’t already, it’s time to start exploring the world of sets in PHP and take your coding skills to the next level.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |