In computer science, a Set is a data structure that stores a collection of unique elements. It is typically implemented as an unordered list of elements, where each element can only appear once. Sets are useful for a variety of applications, such as removing duplicates from a list, checking for membership of an element, and performing set operations such as union, intersection, and difference. Sets can be implemented using various data structures, such as hash tables, binary search trees, or arrays. Keep reading below to learn how to use a Set in TypeScript.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
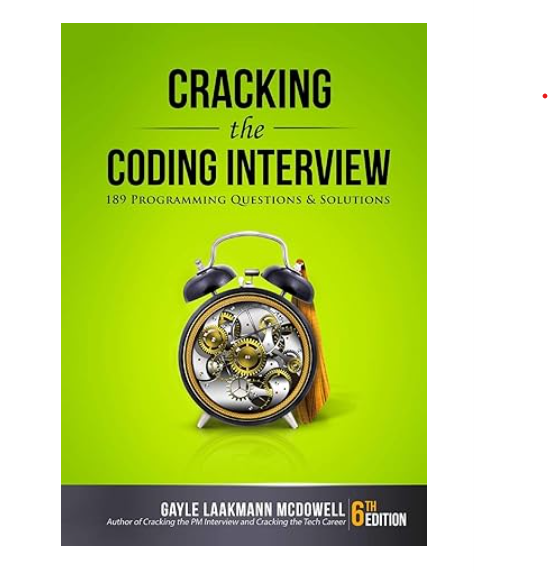
How to use a Set in TypeScript with example code
A Set is a built-in object in TypeScript that allows you to store unique values of any type. In this blog post, we will explore how to use a Set in TypeScript with example code.
To create a new Set in TypeScript, you can use the following syntax:
const mySet = new Set();
This will create an empty Set. You can also initialize a Set with an array of values:
const mySet = new Set([1, 2, 3]);
To add a new value to a Set, you can use the add()
method:
mySet.add(4);
To check if a value exists in a Set, you can use the has()
method:
mySet.has(4); // returns true
To remove a value from a Set, you can use the delete()
method:
mySet.delete(4);
To get the size of a Set, you can use the size
property:
mySet.size; // returns 3
You can also iterate over the values in a Set using a for...of
loop:
for (const value of mySet) {
console.log(value);
}
This will log each value in the Set to the console.
In conclusion, Sets are a useful data structure in TypeScript for storing unique values. They provide methods for adding, removing, and checking if a value exists in the Set. They can also be iterated over using a for...of
loop.
What is a Set in TypeScript?
In conclusion, a Set in TypeScript is a data structure that allows you to store unique values of any type. It is similar to an array, but with the added benefit of automatically removing duplicates. Sets are useful when you need to keep track of a collection of items without worrying about duplicates or order. TypeScript provides built-in support for Sets, making it easy to use them in your code. By understanding the basics of Sets in TypeScript, you can take advantage of this powerful data structure to simplify your code and improve its performance.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |