A socket is a software abstraction that represents an endpoint of a two-way communication link between two programs running on a network. It is a fundamental building block of network programming and allows programs to send and receive data over a network. Sockets can be used for various types of communication protocols, such as TCP, UDP, and HTTP. They provide a simple and flexible interface for network communication and are widely used in client-server applications, web servers, and other network-based systems. Keep reading below to learn how to use a Socket in Bash.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
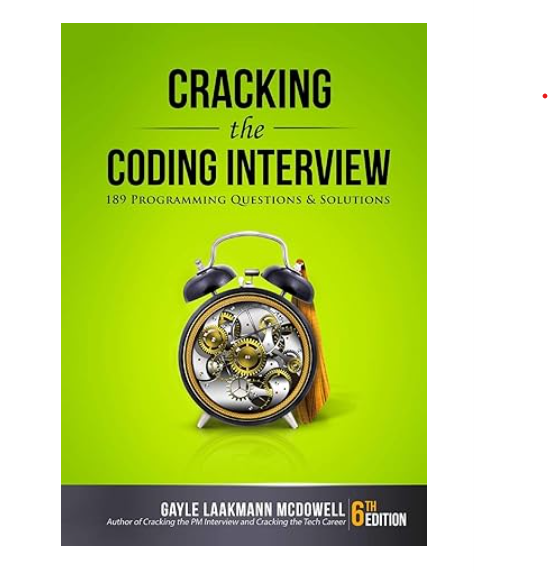
How to use a Socket in Bash with example code
Sockets are a powerful tool for communication between processes. In Bash, we can use sockets to communicate between scripts or even between different instances of Bash. In this post, we will explore how to use sockets in Bash with an example code.
To create a socket in Bash, we can use the `mkfifo` command. This command creates a named pipe, which can be used as a socket. Here is an example of how to create a socket:
mkfifo mysocket
This command creates a named pipe called `mysocket`. We can now use this named pipe to communicate between processes.
To write to the socket, we can use the `echo` command. Here is an example of how to write to the socket:
echo "Hello, world!" > mysocket
This command writes the string “Hello, world!” to the `mysocket` named pipe.
To read from the socket, we can use the `cat` command. Here is an example of how to read from the socket:
cat mysocket
This command reads from the `mysocket` named pipe and outputs the contents to the console.
We can also use sockets to communicate between different instances of Bash. For example, we can create a server script that listens for connections on a socket, and a client script that connects to the server and sends data. Here is an example of a server script:
#!/bin/bash
# Create the socket
mkfifo mysocket
# Listen for connections
while true; do
cat mysocket | while read line; do
echo "Received: $line"
done
done
This script creates a named pipe called `mysocket` and listens for connections. When a connection is made, it reads from the named pipe and outputs the contents to the console.
Here is an example of a client script that connects to the server and sends data:
#!/bin/bash
# Connect to the server
exec 3<> mysocket
# Send data
echo "Hello, server!" >&3
# Close the connection
exec 3>&-
This script connects to the `mysocket` named pipe and sends the string “Hello, server!” to the server. It then closes the connection.
In conclusion, sockets are a powerful tool for communication between processes in Bash. We can use the `mkfifo` command to create named pipes that can be used as sockets. We can then use the `echo` and `cat` commands to write and read from the sockets. We can also use sockets to communicate between different instances of Bash, as demonstrated in the example code.
What is a Socket in Bash?
In conclusion, a socket in Bash is a communication endpoint that allows two processes to communicate with each other over a network. It provides a reliable and efficient way for processes to exchange data, and is commonly used in client-server applications. Sockets can be created and managed using various Bash commands and utilities, such as `nc`, `socat`, and `netcat`. Understanding how sockets work and how to use them effectively can greatly enhance your ability to develop and deploy networked applications in Bash. Whether you are a seasoned Bash developer or just starting out, mastering the use of sockets is an essential skill that can help you build robust and scalable networked applications.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |