A socket is a software endpoint that enables communication between two processes over a network. It is a fundamental data structure in computer networking that allows programs to send and receive data across a network. Sockets provide a standardized interface for network communication, allowing applications to communicate with each other regardless of the underlying network hardware and protocols. They are used extensively in client-server applications, where a client program connects to a server program using a socket, and then sends and receives data over the network. Sockets can be implemented in various programming languages and operating systems, and are an essential component of modern networked computing. Keep reading below to learn how to use a Socket in Python.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
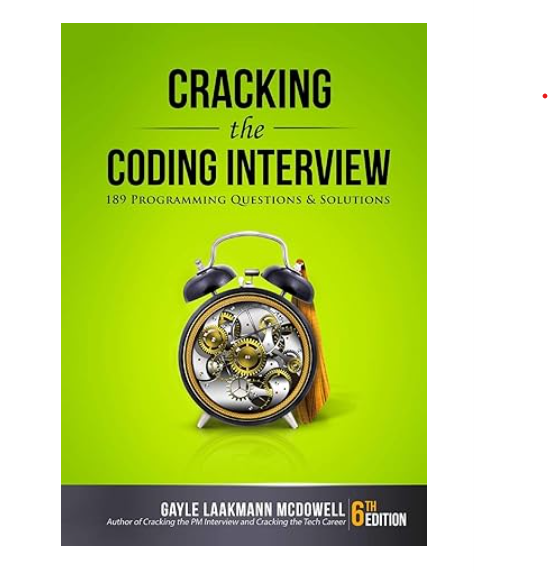
How to use a Socket in Python with example code
Sockets are a fundamental concept in network programming. They are used to establish a connection between two computers over a network. In Python, the `socket` module provides a way to create and use sockets.
To create a socket in Python, you first need to import the `socket` module. Once you have imported the module, you can create a socket object using the `socket()` function. The `socket()` function takes two arguments: the address family and the socket type.
The address family specifies the type of address that the socket can communicate with. The two most common address families are `AF_INET` (IPv4) and `AF_INET6` (IPv6).
The socket type specifies the type of communication protocol that the socket will use. The two most common socket types are `SOCK_STREAM` (TCP) and `SOCK_DGRAM` (UDP).
Here is an example of how to create a socket in Python:
import socket
# create a socket object
s = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
Once you have created a socket object, you can use it to establish a connection with another computer over a network. To do this, you need to call the `connect()` method on the socket object and pass in the address of the computer you want to connect to.
Here is an example of how to establish a connection with a remote computer using a socket in Python:
import socket
# create a socket object
s = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
# connect to a remote computer
s.connect(("www.google.com", 80))
In this example, we are connecting to the Google website on port 80 (the default port for HTTP).
Once you have established a connection, you can send and receive data using the socket object. To send data, you can use the `send()` method on the socket object. To receive data, you can use the `recv()` method on the socket object.
Here is an example of how to send and receive data using a socket in Python:
import socket
# create a socket object
s = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
# connect to a remote computer
s.connect(("www.google.com", 80))
# send a request to the remote computer
s.send(b"GET / HTTP/1.1\r\nHost: www.google.com\r\n\r\n")
# receive data from the remote computer
data = s.recv(1024)
# print the data
print(data)
In this example, we are sending an HTTP request to the Google website and receiving the response. The `b` before the string indicates that it should be sent as bytes.
Overall, sockets are a powerful tool for network programming in Python. With the `socket` module, you can create and use sockets to establish connections with other computers over a network and send and receive data.
What is a Socket in Python?
In conclusion, a socket in Python is a powerful tool that allows for communication between different devices over a network. It provides a way for programs to send and receive data, making it an essential component of many network-based applications. With Python’s built-in socket module, developers can easily create and manage sockets, allowing them to build robust and reliable network applications. Whether you’re building a chat application, a file transfer system, or any other network-based program, understanding sockets in Python is a crucial skill to have. So, if you’re looking to develop network-based applications, be sure to learn about sockets in Python and take advantage of their capabilities.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |