A stack is a linear data structure that follows the Last-In-First-Out (LIFO) principle, where the last element added to the stack is the first one to be removed. It consists of two main operations: push, which adds an element to the top of the stack, and pop, which removes the top element from the stack. Other operations include peek, which returns the top element without removing it, and isEmpty, which checks if the stack is empty. Stacks are commonly used in programming languages for function calls, expression evaluation, and memory management. Keep reading below to learn how to use a Stack in Rust.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
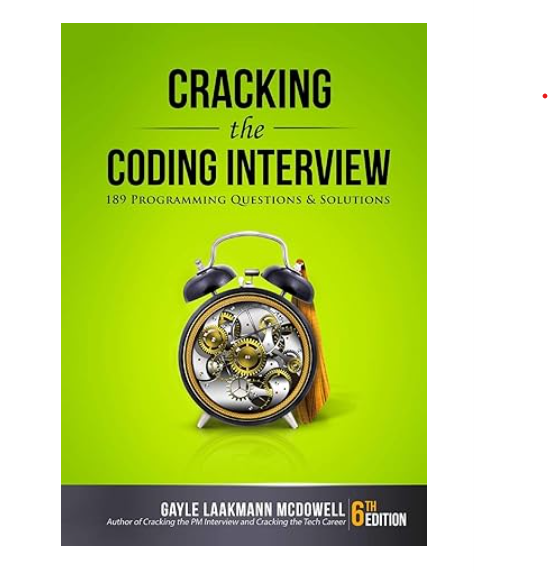
How to use a Stack in Rust with example code
Stacks are a fundamental data structure in computer science that follow the Last-In-First-Out (LIFO) principle. In Rust, we can implement a stack using the built-in `Vec` type.
To use a stack in Rust, we first need to create a new `Vec` and push elements onto it using the `push` method. We can then pop elements off the stack using the `pop` method. Here’s an example:
let mut stack = Vec::new();
stack.push(1);
stack.push(2);
stack.push(3);
assert_eq!(stack.pop(), Some(3));
assert_eq!(stack.pop(), Some(2));
assert_eq!(stack.pop(), Some(1));
assert_eq!(stack.pop(), None);
In this example, we create a new `Vec` called `stack` and push the integers 1, 2, and 3 onto it. We then use the `pop` method to remove the elements from the stack in reverse order.
Note that we need to use `mut` to make the `stack` variable mutable, since we’re modifying it by pushing and popping elements.
Overall, using a stack in Rust is straightforward thanks to the `Vec` type. By following the LIFO principle, we can easily manage collections of data in our Rust programs.
What is a Stack in Rust?
In conclusion, a stack is a fundamental data structure in computer science that is used to store and manage data in a specific way. In Rust, a stack is implemented as a Last-In-First-Out (LIFO) structure, meaning that the last item added to the stack is the first one to be removed. Rust’s ownership and borrowing system ensures that the stack is managed efficiently and safely, preventing common issues such as memory leaks and data races. By understanding the basics of stacks in Rust, developers can create more efficient and reliable programs that can handle complex data structures with ease. Whether you are a beginner or an experienced Rust developer, understanding the stack is essential for building robust and scalable applications.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |