A stack is a linear data structure that follows the Last-In-First-Out (LIFO) principle, where the last element added to the stack is the first one to be removed. It consists of two main operations: push, which adds an element to the top of the stack, and pop, which removes the top element from the stack. Additionally, there is a peek operation that allows you to view the top element without removing it. Stacks are commonly used in programming languages for function calls, as well as in algorithms such as depth-first search and backtracking. Keep reading below to learn how to use a Stack in TypeScript.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
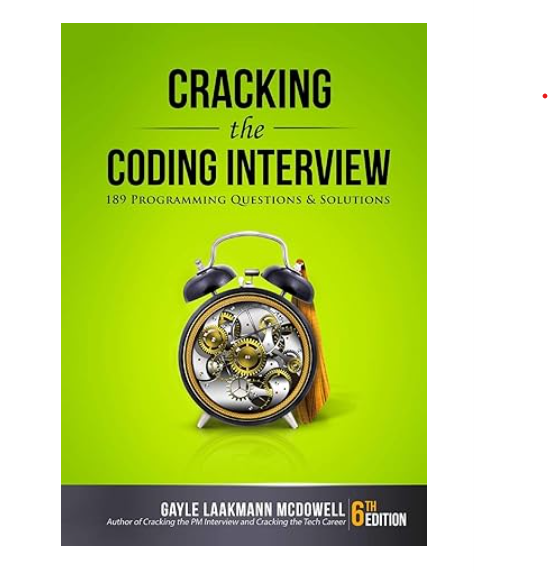
How to use a Stack in TypeScript with example code
A Stack is a data structure that follows the Last-In-First-Out (LIFO) principle. In TypeScript, we can implement a Stack using an array and some built-in array methods.
To create a Stack class in TypeScript, we can define a class with an array property and methods to push, pop, and peek the top element of the stack. Here’s an example implementation:
class Stack
private items: T[] = [];
push(item: T) {
this.items.push(item);
}
pop(): T | undefined {
return this.items.pop();
}
peek(): T | undefined {
return this.items[this.items.length - 1];
}
isEmpty(): boolean {
return this.items.length === 0;
}
size(): number {
return this.items.length;
}
}
In this example, we define a generic Stack class that can hold any type of data. The class has a private array property called items, which holds the elements of the stack. We also define methods to push, pop, and peek the top element of the stack, as well as methods to check if the stack is empty and to get the size of the stack.
To use the Stack class, we can create a new instance and call its methods. Here’s an example:
const stack = new Stack
stack.push(1);
stack.push(2);
stack.push(3);
console.log(stack.peek()); // Output: 3
console.log(stack.pop()); // Output: 3
console.log(stack.size()); // Output: 2
In this example, we create a new Stack instance that can hold numbers. We push three numbers onto the stack, then peek at the top element (which is 3), pop the top element (which is also 3), and get the size of the stack (which is 2).
Using a Stack can be useful in many situations, such as parsing expressions, implementing undo/redo functionality, and solving certain types of problems in computer science. With TypeScript, we can easily create and use a Stack class to handle these situations.
What is a Stack in TypeScript?
In conclusion, a Stack in TypeScript is a data structure that follows the Last-In-First-Out (LIFO) principle. It allows for efficient storage and retrieval of data, making it a valuable tool for developers working on complex applications. With TypeScript, developers can take advantage of the language’s strong typing and object-oriented features to create robust and reliable stacks. Whether you’re building a web application or a mobile app, understanding the basics of a Stack in TypeScript can help you write more efficient and effective code. So, if you’re looking to improve your TypeScript skills, learning about Stacks is a great place to start.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |