Subprocess is a data structure in computer science that allows a program to spawn new processes and communicate with them. It is commonly used in operating systems to manage multiple tasks and programs simultaneously. Subprocesses can be created to run in the background or foreground, and can be used to execute commands, run scripts, or perform other tasks. Communication between subprocesses can be achieved through various mechanisms such as pipes, sockets, or shared memory. Subprocesses are an important tool for building complex systems and applications that require parallel processing and inter-process communication. Keep reading below to learn how to use a Subrocess in C#.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
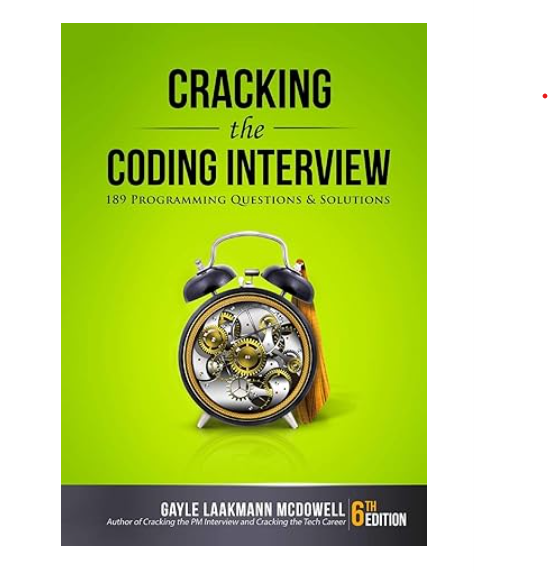
How to use a Subrocess in C# with example code
Subprocesses are a powerful tool in C# that allow you to execute external programs or commands from within your code. This can be useful for a variety of tasks, such as running shell commands, launching other applications, or executing scripts. In this blog post, we will explore how to use subprocesses in C# and provide some example code to get you started.
To use subprocesses in C#, you will need to use the Process class, which is part of the System.Diagnostics namespace. This class provides a way to start and control external processes from within your code. Here is an example of how to use the Process class to execute a shell command:
Process process = new Process();
process.StartInfo.FileName = "cmd.exe";
process.StartInfo.Arguments = "/c dir";
process.StartInfo.UseShellExecute = false;
process.StartInfo.RedirectStandardOutput = true;
process.Start();
string output = process.StandardOutput.ReadToEnd();
process.WaitForExit();
In this example, we create a new Process object and set its FileName property to “cmd.exe”, which is the command prompt on Windows. We also set the Arguments property to “/c dir”, which tells the command prompt to execute the “dir” command. We then set the UseShellExecute property to false and RedirectStandardOutput to true, which allows us to capture the output of the command. Finally, we start the process, read its output, and wait for it to exit.
You can also use the Process class to launch other applications from within your code. Here is an example of how to launch Notepad:
Process.Start("notepad.exe");
This code simply calls the static Start method of the Process class and passes in the name of the application to launch.
In conclusion, subprocesses are a powerful tool in C# that allow you to execute external programs or commands from within your code. The Process class provides a way to start and control external processes, and can be used for a variety of tasks. We hope this blog post has been helpful in getting you started with subprocesses in C#.
What is a Subrocess in C#?
In conclusion, a subprocess in C# is a separate process that is created and managed by the main process. It allows for the execution of multiple tasks simultaneously, improving the overall efficiency and performance of the application. Subprocesses can be used for a variety of purposes, such as running background tasks, handling user input, and communicating with external systems. By understanding the concept of subprocesses in C#, developers can create more robust and scalable applications that can handle complex tasks with ease. Overall, subprocesses are an essential tool for any C# developer looking to optimize their code and improve the user experience.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |