Subprocess is a data structure in computer science that allows a program to spawn new processes and communicate with them. It is commonly used in operating systems to manage multiple tasks simultaneously. Subprocesses can be created to run in the background while the main program continues to execute, or they can be used to execute external commands and retrieve their output. Subprocesses can also be used to manage resources and handle errors in a program. Overall, subprocesses are a powerful tool for managing complex programs and improving their performance. Keep reading below to learn how to use a Subrocess in Kotlin.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
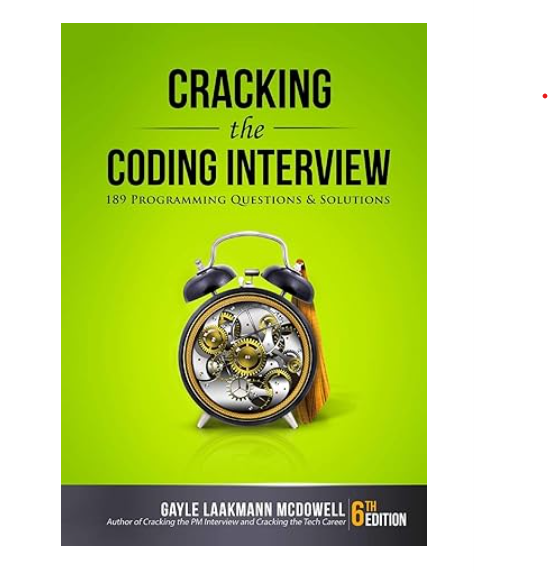
How to use a Subrocess in Kotlin with example code
Subprocesses are a powerful tool in programming that allow you to execute external commands or programs from within your code. In Kotlin, you can use the `ProcessBuilder` class to create and manage subprocesses.
To create a subprocess, you first need to create a `ProcessBuilder` object and specify the command you want to execute. For example, if you want to execute the `ls` command in a Unix-based system, you can create a `ProcessBuilder` object like this:
val processBuilder = ProcessBuilder("ls")
Once you have created the `ProcessBuilder` object, you can start the subprocess by calling the `start()` method:
val process = processBuilder.start()
This will start the subprocess and return a `Process` object that you can use to interact with the subprocess. For example, you can read the output of the subprocess by getting the `InputStream` of the subprocess using the `getInputStream()` method:
val inputStream = process.inputStream
You can then read the output of the subprocess by reading from the `InputStream`. Here is an example of how to read the output of the `ls` command:
val reader = BufferedReader(InputStreamReader(inputStream))
var line: String?
while (reader.readLine().also { line = it } != null) {
println(line)
}
This will print out the output of the `ls` command to the console.
You can also interact with the subprocess by writing to its `OutputStream` using the `getOutputStream()` method. For example, if you want to execute the `echo` command with an argument, you can create a `ProcessBuilder` object like this:
val processBuilder = ProcessBuilder("echo", "Hello, world!")
You can then start the subprocess and write to its `OutputStream` like this:
val process = processBuilder.start()
val outputStream = process.outputStream
val writer = BufferedWriter(OutputStreamWriter(outputStream))
writer.write("Hello, subprocess!")
writer.newLine()
writer.flush()
This will execute the `echo` command with the argument “Hello, world!” and write “Hello, subprocess!” to its `OutputStream`.
In conclusion, subprocesses are a powerful tool in programming that allow you to execute external commands or programs from within your code. In Kotlin, you can use the `ProcessBuilder` class to create and manage subprocesses. You can interact with the subprocess by reading from its `InputStream` and writing to its `OutputStream`.
What is a Subrocess in Kotlin?
In conclusion, a subprocess in Kotlin is a way to break down a larger process into smaller, more manageable parts. By dividing a complex task into smaller sub-tasks, developers can write more efficient and maintainable code. Subprocesses in Kotlin can be implemented using functions, coroutines, or other programming constructs. They allow developers to write code that is easier to understand, test, and debug. By using subprocesses, developers can improve the overall quality of their code and make it more scalable and adaptable to changing requirements. In short, subprocesses are an essential tool for any Kotlin developer looking to write clean, efficient, and maintainable code.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |