Subprocess is a data structure in computer science that allows a program to spawn new processes and communicate with them. It is commonly used in operating systems to manage multiple tasks simultaneously. Subprocesses can be created to run in the background while the main program continues to execute, allowing for efficient use of system resources. Communication between the main program and subprocesses can be achieved through various mechanisms such as pipes, sockets, and shared memory. Subprocesses can also be used to execute external programs and scripts, making it a powerful tool for automation and integration. Keep reading below to learn how to use a Subrocess in TypeScript.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
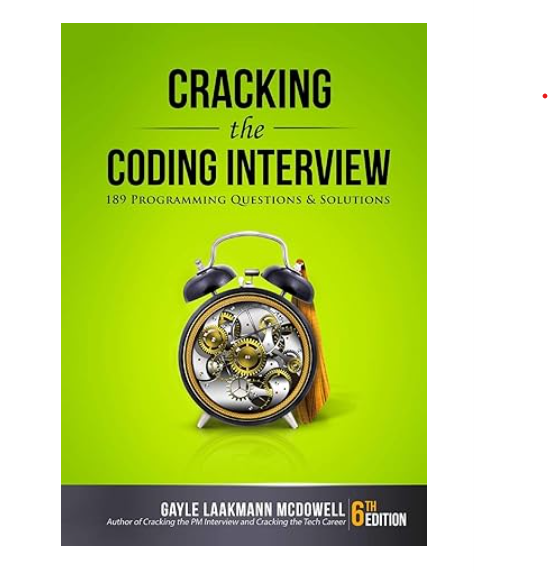
How to use a Subrocess in TypeScript with example code
Subprocesses are a powerful tool in programming that allow you to run external commands or scripts from within your code. In TypeScript, you can use the built-in `child_process` module to create and manage subprocesses.
To create a subprocess in TypeScript, you first need to import the `spawn` function from the `child_process` module. This function takes two arguments: the command to run, and an array of arguments to pass to the command. For example, to run the `ls` command in a subprocess and print the output to the console, you could use the following code:
import { spawn } from 'child_process';
const ls = spawn('ls', ['-lh', '/usr']);
ls.stdout.on('data', (data) => {
console.log(`stdout: ${data}`);
});
ls.stderr.on('data', (data) => {
console.error(`stderr: ${data}`);
});
ls.on('close', (code) => {
console.log(`child process exited with code ${code}`);
});
In this example, we create a subprocess that runs the `ls` command with the `-lh` and `/usr` arguments. We then listen for events on the subprocess’s `stdout`, `stderr`, and `close` streams. When data is received on the `stdout` or `stderr` streams, we print it to the console. When the subprocess exits, we print its exit code.
Subprocesses can be used for a wide variety of tasks, from running shell scripts to executing external programs. By using TypeScript’s `child_process` module, you can easily create and manage subprocesses in your code.
What is a Subrocess in TypeScript?
In conclusion, a subprocess in TypeScript is a function that is called within another function. It allows for the separation of concerns and the creation of reusable code. By breaking down a larger function into smaller subprocesses, developers can improve the readability and maintainability of their code. Additionally, subprocesses can be used to handle specific tasks or operations, making the overall function more efficient and effective. Overall, understanding and utilizing subprocesses in TypeScript can greatly enhance the development process and lead to more robust and scalable applications.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |