In computer science, a thread is a unit of execution within a process. A process can have multiple threads, each of which can run concurrently and independently of each other. Threads share the same memory space and resources of the process they belong to, but have their own stack and program counter. Threads are commonly used in multi-threaded applications to improve performance and responsiveness, as well as to simplify programming by allowing multiple tasks to be performed simultaneously within a single process. However, managing threads can be complex and requires careful synchronization to avoid race conditions and other concurrency issues. Keep reading below to learn how to use a Thread in Java.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
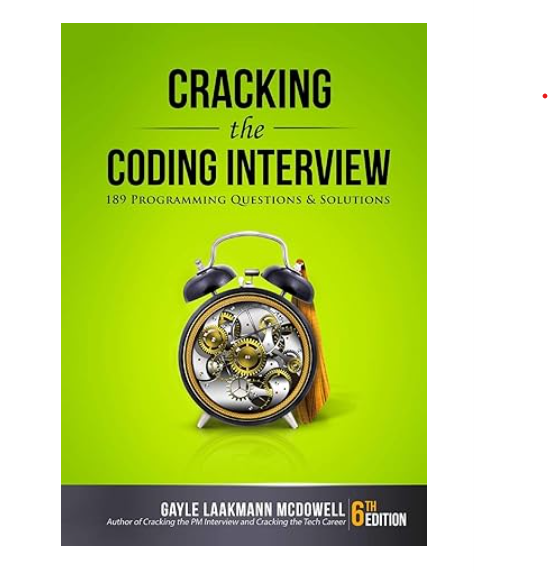
How to use a Thread in Java with example code
Java provides a way to execute multiple threads concurrently. A thread is a lightweight sub-process, and multiple threads can run in parallel within a single program. In this blog post, we will discuss how to use a Thread in Java with example code.
To create a new thread, we need to extend the Thread class or implement the Runnable interface. The Runnable interface provides a run() method that needs to be implemented. The run() method contains the code that will be executed when the thread is started.
Here is an example of extending the Thread class:
class MyThread extends Thread {
public void run() {
System.out.println("MyThread is running");
}
}
To start the thread, we need to create an instance of the MyThread class and call the start() method:
MyThread myThread = new MyThread();
myThread.start();
The start() method creates a new thread and calls the run() method.
Here is an example of implementing the Runnable interface:
class MyRunnable implements Runnable {
public void run() {
System.out.println("MyRunnable is running");
}
}
To start the thread, we need to create an instance of the MyRunnable class and pass it to a Thread object:
MyRunnable myRunnable = new MyRunnable();
Thread thread = new Thread(myRunnable);
thread.start();
The start() method creates a new thread and calls the run() method of the MyRunnable object.
In conclusion, using threads in Java can help improve the performance of our programs by allowing multiple tasks to run concurrently. We can create a new thread by extending the Thread class or implementing the Runnable interface. We then need to call the start() method to start the thread and execute the code in the run() method.
What is a Thread in Java?
In conclusion, a Thread in Java is a lightweight process that enables concurrent execution of multiple tasks within a single program. Threads allow for efficient use of system resources and can greatly improve the performance of a program. Java provides a rich set of APIs for creating and managing threads, including synchronization mechanisms to ensure thread safety. Understanding how to use threads effectively is an important skill for any Java developer, as it can help to create more responsive and scalable applications. By leveraging the power of threads, developers can build complex and sophisticated applications that can handle multiple tasks simultaneously, without sacrificing performance or stability.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |