In computer science, a thread is a unit of execution within a process. A process can have multiple threads, each of which can run concurrently and independently of each other. Threads share the same memory space and resources of the process they belong to, but have their own stack and program counter. Threads are commonly used in multi-threaded applications to improve performance and responsiveness, as well as to simplify programming by allowing multiple tasks to be performed simultaneously within a single process. However, managing threads can be complex and requires careful synchronization to avoid race conditions and other concurrency issues. Keep reading below to learn how to use a Thread in Kotlin.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
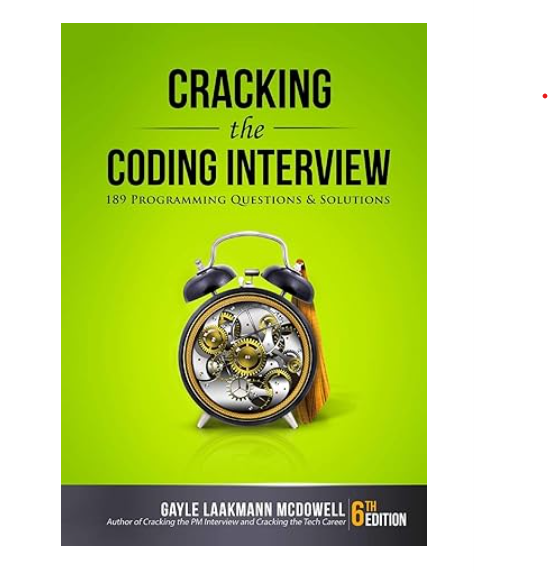
How to use a Thread in Kotlin with example code
Threads are an essential part of concurrent programming. They allow multiple tasks to run simultaneously, which can improve the performance of your application. In Kotlin, you can create a thread using the `Thread` class.
To create a thread, you need to create an instance of the `Thread` class and pass a lambda expression to its constructor. The lambda expression should contain the code that you want to run in the thread. Here’s an example:
val thread = Thread {
// Code to be executed in the thread
}
Once you have created the thread, you can start it by calling the `start()` method. This will execute the code in the lambda expression in a separate thread. Here’s an example:
val thread = Thread {
// Code to be executed in the thread
}
thread.start()
You can also pass parameters to the lambda expression using the `Thread` constructor. Here’s an example:
val thread = Thread({
// Code to be executed in the thread
}, "Thread Name")
thread.start()
In this example, we have passed a string parameter to the lambda expression and also specified a name for the thread.
It’s important to note that when you create a thread, it runs independently of the main thread. This means that the code in the main thread and the code in the thread can run simultaneously. If you need to wait for the thread to finish before continuing with the main thread, you can use the `join()` method. Here’s an example:
val thread = Thread {
// Code to be executed in the thread
}
thread.start()
thread.join()
In this example, the `join()` method is called after the `start()` method. This will cause the main thread to wait for the thread to finish before continuing.
In conclusion, threads are a powerful tool for concurrent programming in Kotlin. By using the `Thread` class, you can create and manage threads in your application.
What is a Thread in Kotlin?
In conclusion, a thread in Kotlin is a lightweight unit of execution that allows for concurrent programming. It enables multiple tasks to be executed simultaneously, improving the performance and responsiveness of an application. Kotlin provides built-in support for creating and managing threads through the use of the Thread class and the kotlinx.coroutines library. With the ability to run tasks in parallel, developers can create more efficient and responsive applications that can handle complex operations without blocking the main thread. Understanding how to use threads in Kotlin is an essential skill for any developer looking to create high-performance applications.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |