A tree is a hierarchical data structure in computer science that consists of nodes connected by edges. Each node in a tree has a parent node and zero or more child nodes. The topmost node in a tree is called the root node, and the nodes at the bottom of the tree that have no children are called leaf nodes. Trees are commonly used to represent hierarchical relationships between data, such as file systems, organization charts, and family trees. They are also used in algorithms such as binary search trees and heap data structures. Keep reading below to learn how to use a Tree in Kotlin.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
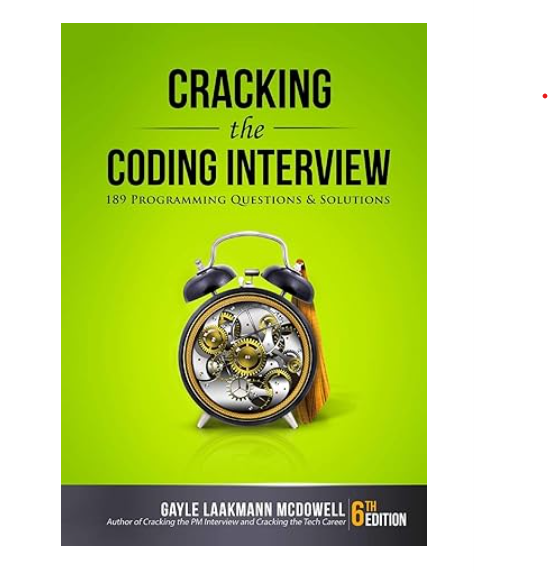
How to use a Tree in Kotlin with example code
A Tree is a data structure that represents a hierarchical structure. It consists of nodes that are connected by edges. Each node can have zero or more child nodes. In this blog post, we will learn how to use a Tree in Kotlin with example code.
To create a Tree in Kotlin, we can define a Node class that represents a node in the Tree. The Node class can have a value and a list of child nodes. Here is an example code:
class Node(val value: Int, val children: MutableList
In this example, the Node class has a constructor that takes a value and a list of child nodes. The list of child nodes is initialized to an empty mutable list by default.
To add a child node to a Node, we can define a method that takes a Node as a parameter and adds it to the list of child nodes. Here is an example code:
fun addChild(node: Node) {
children.add(node)
}
In this example, the addChild method takes a Node as a parameter and adds it to the list of child nodes.
To traverse a Tree, we can define a recursive function that visits each node in the Tree. Here is an example code:
fun traverse(node: Node) {
println(node.value)
for (child in node.children) {
traverse(child)
}
}
In this example, the traverse function takes a Node as a parameter and prints its value. Then, it recursively calls itself for each child node.
To create a Tree, we can create a root Node and add child nodes to it. Here is an example code:
val root = Node(1)
val child1 = Node(2)
val child2 = Node(3)
val child3 = Node(4)
root.addChild(child1)
root.addChild(child2)
child2.addChild(child3)
traverse(root)
In this example, we create a root Node with a value of 1. Then, we create three child nodes with values of 2, 3, and 4. We add child1 and child2 to the root Node, and child3 to child2. Finally, we call the traverse function with the root Node to print the values of all nodes in the Tree.
In conclusion, a Tree is a useful data structure for representing hierarchical structures. In Kotlin, we can create a Tree by defining a Node class and adding child nodes to it. We can traverse a Tree by defining a recursive function that visits each node in the Tree.
What is a Tree in Kotlin?
In conclusion, a tree in Kotlin is a data structure that consists of nodes connected by edges. Each node can have zero or more child nodes, and the topmost node is called the root. Trees are commonly used in computer science and programming to represent hierarchical relationships between data. In Kotlin, trees can be implemented using classes and objects, and there are various algorithms and operations that can be performed on them. Understanding the concept of trees and how to work with them in Kotlin can be a valuable skill for any programmer.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |