A tree is a hierarchical data structure in computer science that consists of nodes connected by edges. Each node in a tree has a parent node and zero or more child nodes. The topmost node in a tree is called the root node, and the nodes at the bottom of the tree are called leaf nodes. Trees are commonly used to represent hierarchical relationships between data, such as file systems, organization charts, and family trees. They are also used in algorithms such as binary search trees and heap data structures. Keep reading below to learn how to use a Tree in TypeScript.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
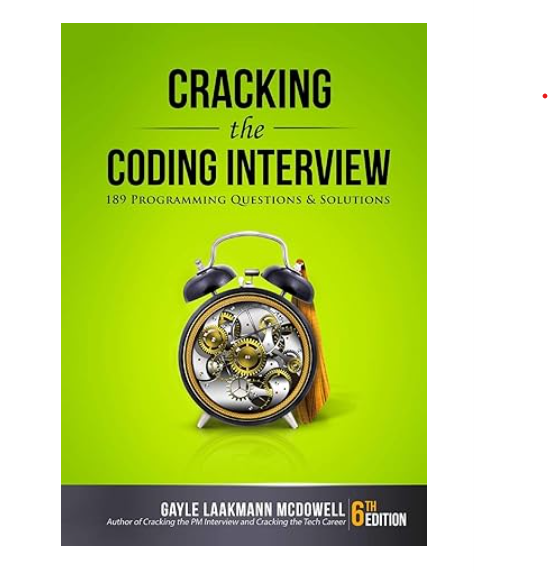
How to use a Tree in TypeScript with example code
Trees are a fundamental data structure in computer science and are used to represent hierarchical relationships between data. In TypeScript, we can implement a tree using classes and interfaces.
To create a tree, we first define a `Node` class that represents a single node in the tree. Each node has a value and an array of child nodes.
“`typescript
class Node
value: T;
children: Node
constructor(value: T) {
this.value = value;
this.children = [];
}
addChild(node: Node
this.children.push(node);
}
}
“`
Next, we define a `Tree` class that represents the entire tree. The `Tree` class has a single property, the root node of the tree.
“`typescript
class Tree
root: Node
constructor(rootValue: T) {
this.root = new Node(rootValue);
}
}
“`
To use the tree, we can create a new instance of the `Tree` class and add nodes to it.
“`typescript
const tree = new Tree
const child1 = new Node(‘child1’);
const child2 = new Node(‘child2’);
const grandchild1 = new Node(‘grandchild1’);
const grandchild2 = new Node(‘grandchild2’);
tree.root.addChild(child1);
tree.root.addChild(child2);
child1.addChild(grandchild1);
child2.addChild(grandchild2);
“`
In this example, we create a tree with a root node that has two child nodes. Each child node has a single grandchild node.
We can traverse the tree using depth-first search or breadth-first search. Here’s an example of a depth-first search implementation:
“`typescript
function depthFirstSearch
visitFn(node);
if (node.children.length) {
node.children.forEach(child => {
depthFirstSearch(child, visitFn);
});
}
}
“`
We can use this function to visit each node in the tree:
“`typescript
depthFirstSearch(tree.root, node => {
console.log(node.value);
});
“`
This will output the values of each node in the tree in depth-first order:
“`
root
child1
grandchild1
child2
grandchild2
“`
What is a Tree in TypeScript?
In conclusion, a Tree in TypeScript is a data structure that consists of nodes connected by edges. Each node can have zero or more child nodes, and the topmost node is called the root node. Trees are commonly used in computer science and programming to represent hierarchical relationships between data. In TypeScript, trees can be implemented using classes and interfaces, and can be used to solve a wide range of problems, from parsing and analyzing data to building user interfaces. By understanding the basics of trees in TypeScript, developers can create more efficient and effective code, and build better software applications.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |