Trie is a tree-like data structure used in computer science to efficiently store and retrieve strings. It is also known as a prefix tree, as it stores strings by breaking them down into their individual characters and organizing them in a tree structure based on their common prefixes. Each node in the tree represents a single character, and the path from the root to a particular node represents a string. Tries are commonly used in applications such as autocomplete, spell checkers, and IP routing tables, as they allow for fast and efficient searching and retrieval of strings. Keep reading below to learn how to use a Trie in C++.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
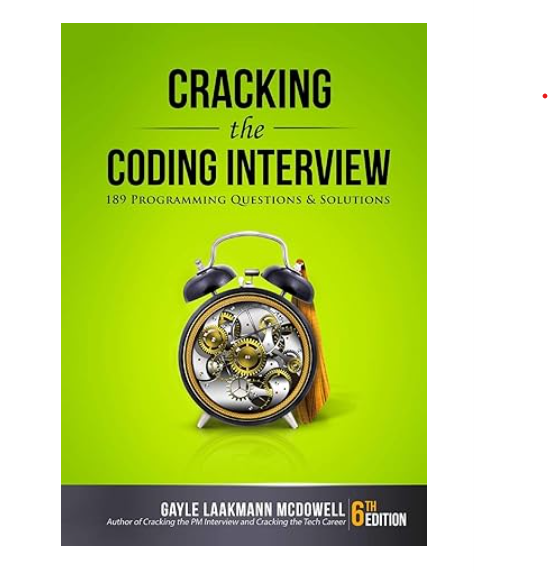
How to use a Trie in C++ with example code
Trie is a tree-like data structure that is used to store a collection of strings. It is also known as a prefix tree because it can efficiently search for all strings that have a given prefix. In this blog post, we will learn how to use a Trie in C++ with example code.
To implement a Trie in C++, we can use a class to represent each node in the tree. Each node will have an array of pointers to its children, and a boolean flag to indicate whether the node represents the end of a string. Here is an example implementation of the TrieNode class:
class TrieNode {
public:
TrieNode* children[26];
bool isEndOfWord;
TrieNode() {
for (int i = 0; i < 26; i++) {
children[i] = nullptr;
}
isEndOfWord = false;
}
};
In this implementation, we assume that we are only dealing with lowercase English letters. Therefore, we use an array of size 26 to store the pointers to the children. Each element in the array corresponds to a letter in the alphabet.
To insert a string into the Trie, we start at the root node and traverse the tree one character at a time. If a child node corresponding to the current character does not exist, we create a new node and add it as a child of the current node. We repeat this process until we reach the end of the string, at which point we set the isEndOfWord flag of the last node to true. Here is an example implementation of the insert function:
void insert(TrieNode* root, string word) {
TrieNode* node = root;
for (char c : word) {
int index = c - 'a';
if (node->children[index] == nullptr) {
node->children[index] = new TrieNode();
}
node = node->children[index];
}
node->isEndOfWord = true;
}
To search for a string in the Trie, we start at the root node and traverse the tree one character at a time. If a child node corresponding to the current character does not exist, we know that the string is not in the Trie. We repeat this process until we reach the end of the string. If the isEndOfWord flag of the last node is true, we know that the string is in the Trie. Here is an example implementation of the search function:
bool search(TrieNode* root, string word) {
TrieNode* node = root;
for (char c : word) {
int index = c - 'a';
if (node->children[index] == nullptr) {
return false;
}
node = node->children[index];
}
return node->isEndOfWord;
}
In conclusion, Trie is a powerful data structure that can be used to efficiently store and search for a collection of strings. In this blog post, we learned how to implement a Trie in C++ and how to use it to insert and search for strings.
What is a Trie in C++?
In conclusion, a Trie is a data structure that is used to efficiently store and search for strings in a computer program. It is particularly useful when dealing with large sets of strings, as it allows for fast lookups and insertions. In C++, Tries can be implemented using a variety of techniques, including arrays, linked lists, and hash tables. By using a Trie, programmers can improve the performance of their programs and make them more efficient. Overall, understanding the basics of Tries is an important skill for any C++ programmer who wants to optimize their code and improve its functionality.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |