Trie is a tree-like data structure used in computer science to efficiently store and retrieve strings. It is also known as a prefix tree, as it stores strings by breaking them down into their individual characters and organizing them in a tree structure based on their common prefixes. Each node in the tree represents a single character, and the path from the root to a leaf node represents a complete string. Tries are commonly used in applications such as autocomplete, spell checkers, and IP routing tables, as they allow for fast and efficient searching and retrieval of strings. Keep reading below to learn how to use a Trie in Go.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
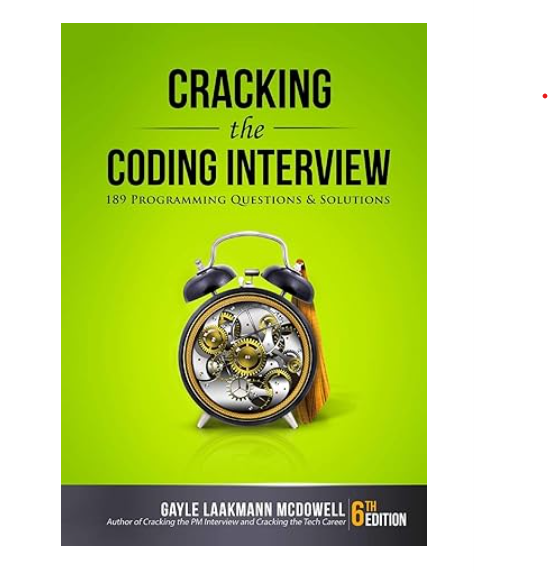
How to use a Trie in Go with example code
Trie, also known as prefix tree, is a tree-like data structure that is commonly used for efficient string searching. In this blog post, we will explore how to use a Trie in Go with example code.
To get started, we need to define the Trie data structure. In Go, we can define a Trie as a struct that contains a map of child nodes and a boolean flag to indicate whether the current node represents the end of a word. Here’s an example:
type TrieNode struct {
children map[rune]*TrieNode
isEnd bool
}
The children
map stores the child nodes of the current node, where the key is a rune (a Unicode code point) and the value is a pointer to the child node. The isEnd
flag is set to true if the current node represents the end of a word.
Next, let’s define some methods for the Trie. The first method is Insert
, which inserts a word into the Trie. Here’s the code:
func (root *TrieNode) Insert(word string) {
node := root
for _, ch := range word {
if node.children[ch] == nil {
node.children[ch] = &TrieNode{children: make(map[rune]*TrieNode)}
}
node = node.children[ch]
}
node.isEnd = true
}
The Insert
method iterates over each character in the word and adds a child node for each character if it doesn’t already exist. Finally, it sets the isEnd
flag of the last node to true.
The next method is Search
, which searches for a word in the Trie. Here’s the code:
func (root *TrieNode) Search(word string) bool {
node := root
for _, ch := range word {
if node.children[ch] == nil {
return false
}
node = node.children[ch]
}
return node.isEnd
}
The Search
method iterates over each character in the word and follows the child nodes. If a child node doesn’t exist for a character, it means the word doesn’t exist in the Trie and the method returns false. If the method reaches the end of the word and the isEnd
flag is true, it means the word exists in the Trie and the method returns true.
Finally, let’s see an example of how to use the Trie. Suppose we want to store a list of words and check if a given word exists in the list. We can create a Trie and insert each word into it, like this:
words := []string{"apple", "banana", "cherry", "orange"}
root := &TrieNode{children: make(map[rune]*TrieNode)}
for _, word := range words {
root.Insert(word)
}
Now, we can search for a word in the Trie using the Search
method, like this:
fmt.Println(root.Search("banana")) // true
fmt.Println(root.Search("grape")) // false
In this example, the Search
method returns true for “banana” because it exists in the Trie, and false for “grape” because it doesn’t exist in the Trie.
That’s it! We’ve learned how to use a Trie in Go with example code. Tries are a powerful data structure for string searching and can be used in many applications, such as autocomplete and spell checking.
What is a Trie in Go?
In conclusion, a Trie is a data structure that is used to efficiently store and retrieve strings. It is particularly useful in scenarios where there is a large number of strings that need to be searched or matched against. In Go, the implementation of a Trie can be achieved using a combination of structs and pointers. By using a Trie, developers can significantly improve the performance of their applications, especially when dealing with large datasets. Overall, understanding the concept of a Trie and how to implement it in Go can be a valuable skill for any developer looking to optimize their code.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |