Trie is a tree-like data structure used in computer science to efficiently store and retrieve strings. It is also known as a prefix tree, as it stores strings by breaking them down into their individual characters and organizing them in a tree structure based on their common prefixes. Each node in the tree represents a single character, and the path from the root to a particular node represents a string. Tries are commonly used in applications such as autocomplete, spell checkers, and IP routing tables, where fast string matching is required. Keep reading below to learn how to use a Trie in Java.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
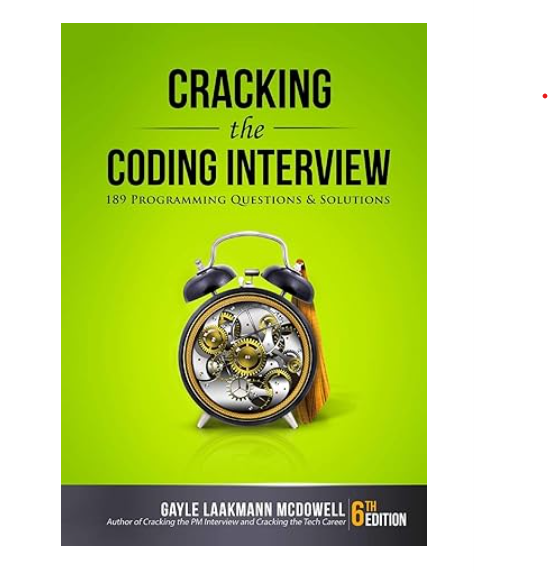
How to use a Trie in Java with example code
Trie is a tree-like data structure that is used to store a collection of strings. It is also known as a prefix tree because it can efficiently search for all strings that have a given prefix. In this blog post, we will learn how to use a Trie in Java with example code.
To implement a Trie in Java, we can use a class to represent each node in the tree. Each node will have a character value and a boolean flag to indicate whether it represents the end of a string. We can also use a HashMap to store the child nodes of each node.
Here is an example implementation of a TrieNode class:
class TrieNode {
char value;
boolean isEndOfWord;
Map<Character, TrieNode> children;
public TrieNode(char value) {
this.value = value;
this.isEndOfWord = false;
this.children = new HashMap<>();
}
}
To insert a string into the Trie, we start at the root node and iterate through each character in the string. For each character, we check if there is a child node with that character value. If there is, we move to that child node. If there isn’t, we create a new child node with the character value and add it to the current node’s children. After iterating through all the characters in the string, we set the isEndOfWord flag of the last node to true.
Here is an example implementation of a Trie class with an insert method:
class Trie {
TrieNode root;
public Trie() {
this.root = new TrieNode('\0');
}
public void insert(String word) {
TrieNode current = root;
for (char c : word.toCharArray()) {
if (!current.children.containsKey(c)) {
current.children.put(c, new TrieNode(c));
}
current = current.children.get(c);
}
current.isEndOfWord = true;
}
}
To search for a string in the Trie, we start at the root node and iterate through each character in the string. For each character, we check if there is a child node with that character value. If there is, we move to that child node. If there isn’t, we know that the string is not in the Trie. After iterating through all the characters in the string, we check if the last node’s isEndOfWord flag is true. If it is, we know that the string is in the Trie.
Here is an example implementation of a search method in the Trie class:
public boolean search(String word) {
TrieNode current = root;
for (char c : word.toCharArray()) {
if (!current.children.containsKey(c)) {
return false;
}
current = current.children.get(c);
}
return current.isEndOfWord;
}
In conclusion, Trie is a powerful data structure that can be used to efficiently store and search for a collection of strings. By implementing a Trie in Java, we can take advantage of its benefits in our applications.
What is a Trie in Java?
In conclusion, a Trie is a data structure that is used to efficiently store and retrieve strings in Java. It is a tree-like structure that allows for fast searching and insertion of strings. Tries are commonly used in applications that require fast string matching, such as spell checkers and search engines. In Java, Tries can be implemented using various techniques, including arrays and linked lists. By using a Trie, developers can improve the performance of their applications and provide a better user experience. Overall, Tries are a powerful tool for managing strings in Java and are worth considering for any application that deals with large amounts of text data.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |