Trie is a tree-like data structure used in computer science to efficiently store and retrieve strings. It is also known as a prefix tree, as it stores strings by breaking them down into their individual characters and organizing them in a tree structure based on their common prefixes. Each node in the tree represents a single character, and the path from the root to a leaf node represents a complete string. Tries are commonly used in applications such as autocomplete, spell checkers, and IP routing tables, as they allow for fast and efficient searching and retrieval of strings. Keep reading below to learn how to use a Trie in Python.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
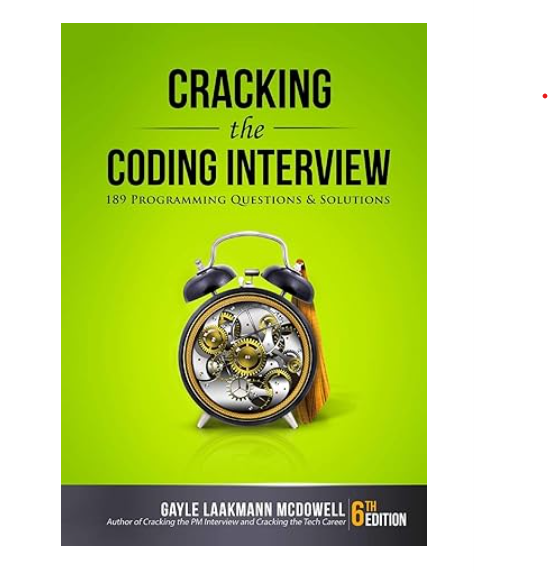
How to use a Trie in Python with example code
Trie is a tree-like data structure that is used to store a collection of strings. It is also known as a prefix tree, as it allows for efficient prefix-based searches. In this blog post, we will explore how to use a Trie in Python.
To implement a Trie in Python, we can use a dictionary to represent each node in the tree. Each key in the dictionary represents a character, and the value is another dictionary representing the children of that node. We can also use a boolean flag to indicate whether a node represents the end of a word.
Let’s take a look at an example implementation of a Trie in Python:
class Trie:
def __init__(self):
self.root = {}
def insert(self, word):
node = self.root
for char in word:
if char not in node:
node[char] = {}
node = node[char]
node['$'] = True
def search(self, word):
node = self.root
for char in word:
if char not in node:
return False
node = node[char]
return '$' in node
def starts_with(self, prefix):
node = self.root
for char in prefix:
if char not in node:
return False
node = node[char]
return True
In this implementation, we define a Trie class with three methods: insert, search, and starts_with. The insert method inserts a word into the Trie by iterating over each character in the word and adding a new node to the Trie if it does not already exist. The search method searches for a word in the Trie by iterating over each character in the word and checking if the node exists. The starts_with method checks if any words in the Trie start with a given prefix by iterating over each character in the prefix and checking if the node exists.
Let’s see how we can use this Trie implementation in practice:
trie = Trie()
trie.insert('apple')
trie.insert('banana')
trie.insert('orange')
print(trie.search('apple')) # True
print(trie.search('banana')) # True
print(trie.search('orange')) # True
print(trie.search('grape')) # False
print(trie.starts_with('app')) # True
print(trie.starts_with('ban')) # True
print(trie.starts_with('ora')) # True
print(trie.starts_with('gra')) # False
In this example, we create a new Trie instance and insert three words: ‘apple’, ‘banana’, and ‘orange’. We then use the search method to check if each word exists in the Trie, and the starts_with method to check if any words in the Trie start with a given prefix.
In conclusion, Trie is a powerful data structure that can be used to efficiently store and search for collections of strings. With the implementation we have provided, you can easily use a Trie in your Python projects.
What is a Trie in Python?
In conclusion, a Trie is a data structure that is used to efficiently store and search for strings in Python. It is a tree-like structure where each node represents a character in a string. Tries are particularly useful when dealing with large datasets of strings, as they allow for fast and efficient searching and retrieval of data. In Python, Tries can be implemented using various techniques such as dictionaries or classes. By using Tries, developers can optimize their code and improve the performance of their applications. Overall, Tries are a powerful tool for managing and manipulating strings in Python, and are definitely worth exploring for anyone working with large datasets of strings.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |