Trie is a tree-like data structure used in computer science to efficiently store and retrieve strings. It is also known as a prefix tree, as it stores strings by breaking them down into their individual characters and organizing them in a tree structure based on their common prefixes. Each node in the tree represents a single character, and the path from the root to a leaf node represents a complete string. Tries are commonly used in applications such as autocomplete, spell checkers, and IP routing tables, as they allow for fast and efficient searching and retrieval of strings. Keep reading below to learn how to use a Trie in Rust.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
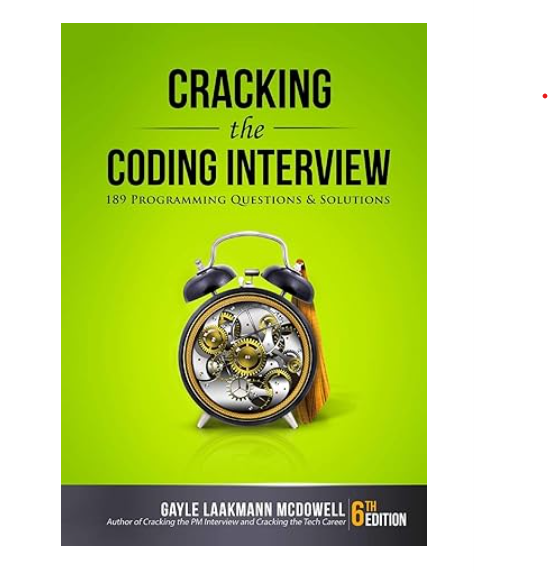
How to use a Trie in Rust with example code
Trie is a data structure that is used to store a set of strings. It is also known as a prefix tree. In this blog post, we will learn how to use a Trie in Rust with example code.
To use a Trie in Rust, we need to first add the `trie` crate to our project. We can do this by adding the following line to our `Cargo.toml` file:
[dependencies]
trie = "0.3.0"
Once we have added the `trie` crate to our project, we can create a new Trie by calling the `Trie::new()` function. We can then insert strings into the Trie using the `insert()` function.
use trie::Trie;
fn main() {
let mut trie = Trie::new();
trie.insert("hello");
trie.insert("world");
}
We can also check if a string exists in the Trie using the `contains()` function.
use trie::Trie;
fn main() {
let mut trie = Trie::new();
trie.insert("hello");
trie.insert("world");
if trie.contains("hello") {
println!("'hello' exists in the Trie");
}
}
We can also remove a string from the Trie using the `remove()` function.
use trie::Trie;
fn main() {
let mut trie = Trie::new();
trie.insert("hello");
trie.insert("world");
trie.remove("hello");
if !trie.contains("hello") {
println!("'hello' does not exist in the Trie");
}
}
In conclusion, Trie is a useful data structure for storing a set of strings. With the `trie` crate in Rust, it is easy to use and implement.
What is a Trie in Rust?
In conclusion, a Trie is a data structure that is used to efficiently store and retrieve strings. It is particularly useful in applications that require fast string matching, such as search engines and spell checkers. In Rust, Tries can be implemented using the standard library’s HashMap or using a custom implementation. Rust’s ownership and borrowing system make it easy to write safe and efficient Trie implementations. By using Tries in Rust, developers can improve the performance of their applications and provide a better user experience.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |