When using a new library such as datetime, you can improve you developer effectiveness by being familiar with the most common questions that come up when using the python datetime library. Using the Stack Overflow Data Explorer tool, we’ve determined the top 10 most popular datetime questions & answers by daily views on Stack Overflow to to be familiar with. Check out the top 10 datetime questions & answers below:
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
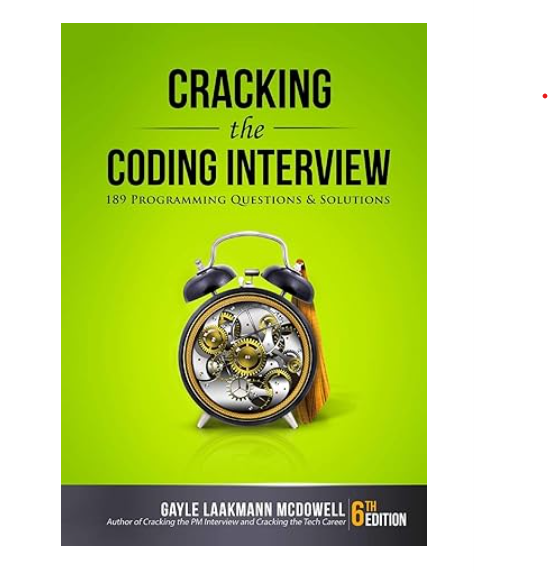
1. How to get the current time in python?
Use:
>>> import datetime
>>> datetime.datetime.now()
datetime.datetime(2009, 1, 6, 15, 8, 24, 78915)
>>> print(datetime.datetime.now())
2009-01-06 15:08:24.789150
And just the time:
>>> datetime.datetime.now().time()
datetime.time(15, 8, 24, 78915)
>>> print(datetime.datetime.now().time())
15:08:24.789150
See the documentation for more information.
To save typing, you can import the datetime
object from the datetime
module:
>>> from datetime import datetime
Then remove the leading datetime.
from all of the above.
2. Converting string into datetime?
datetime.strptime
is the main routine for parsing strings into datetimes. It can handle all sorts of formats, with the format determined by a format string you give it:
from datetime import datetime
datetime_object = datetime.strptime('Jun 1 2005 1:33PM', '%b %d %Y %I:%M%p')
The resulting datetime
object is timezone-naive.
Links:
-
Python documentation for
strptime
/strftime
format strings: Python 2, Python 3 -
strftime.org is also a really nice reference for strftime
Notes:
strptime
= “string parse time”strftime
= “string format time”- Pronounce it out loud today & you won’t have to search for it again in 6 months.
3. Getting today’s date in yyyy-mm-dd in python?
You can use strftime:
>>> from datetime import datetime
>>> datetime.today().strftime('%Y-%m-%d')
'2021-01-26'
Additionally, for anyone also looking for a zero-padded Hour, Minute, and Second at the end: (Comment by Gabriel Staples)
>>> datetime.today().strftime('%Y-%m-%d-%H:%M:%S')
'2021-01-26-16:50:03'
4. Converting unix timestamp string to readable date?
Use datetime
module:
from datetime import datetime
ts = int('1284101485')
# if you encounter a "year is out of range" error the timestamp
# may be in milliseconds, try `ts /= 1000` in that case
print(datetime.utcfromtimestamp(ts).strftime('%Y-%m-%d %H:%M:%S'))
5. How to print a date in a regular format?
The WHY: dates are objects
In Python, dates are objects. Therefore, when you manipulate them, you manipulate objects, not strings or timestamps.
Any object in Python has TWO string representations:
-
The regular representation that is used by
print
can be get using thestr()
function. It is most of the time the most common human readable format and is used to ease display. Sostr(datetime.datetime(2008, 11, 22, 19, 53, 42))
gives you'2008-11-22 19:53:42'
. -
The alternative representation that is used to represent the object nature (as a data). It can be get using the
repr()
function and is handy to know what kind of data your manipulating while you are developing or debugging.repr(datetime.datetime(2008, 11, 22, 19, 53, 42))
gives you'datetime.datetime(2008, 11, 22, 19, 53, 42)'
.
What happened is that when you have printed the date using print
, it used str()
so you could see a nice date string. But when you have printed mylist
, you have printed a list of objects and Python tried to represent the set of data, using repr()
.
The How: what do you want to do with that?
Well, when you manipulate dates, keep using the date objects all long the way. They got thousand of useful methods and most of the Python API expect dates to be objects.
When you want to display them, just use str()
. In Python, the good practice is to explicitly cast everything. So just when it’s time to print, get a string representation of your date using str(date)
.
One last thing. When you tried to print the dates, you printed mylist
. If you want to print a date, you must print the date objects, not their container (the list).
E.G, you want to print all the date in a list :
for date in mylist :
print str(date)
Note that in that specific case, you can even omit str()
because print will use it for you. But it should not become a habit 🙂
Practical case, using your code
import datetime
mylist = []
today = datetime.date.today()
mylist.append(today)
print mylist[0] # print the date object, not the container ;-)
2008-11-22
# It's better to always use str() because :
print "This is a new day : ", mylist[0] # will work
>>> This is a new day : 2008-11-22
print "This is a new day : " + mylist[0] # will crash
>>> cannot concatenate 'str' and 'datetime.date' objects
print "This is a new day : " + str(mylist[0])
>>> This is a new day : 2008-11-22
Advanced date formatting
Dates have a default representation, but you may want to print them in a specific format. In that case, you can get a custom string representation using the strftime()
method.
strftime()
expects a string pattern explaining how you want to format your date.
E.G :
print today.strftime('We are the %d, %b %Y')
>>> 'We are the 22, Nov 2008'
All the letter after a "%"
represent a format for something:
%d
is the day number (2 digits, prefixed with leading zero’s if necessary)%m
is the month number (2 digits, prefixed with leading zero’s if necessary)%b
is the month abbreviation (3 letters)%B
is the month name in full (letters)%y
is the year number abbreviated (last 2 digits)%Y
is the year number full (4 digits)
etc.
Have a look at the official documentation, or McCutchen’s quick reference you can’t know them all.
Since PEP3101, every object can have its own format used automatically by the method format of any string. In the case of the datetime, the format is the same used in
strftime. So you can do the same as above like this:
print "We are the {:%d, %b %Y}".format(today)
>>> 'We are the 22, Nov 2008'
The advantage of this form is that you can also convert other objects at the same time.
With the introduction of Formatted string literals (since Python 3.6, 2016-12-23) this can be written as
import datetime
f"{datetime.datetime.now():%Y-%m-%d}"
>>> '2017-06-15'
Localization
Dates can automatically adapt to the local language and culture if you use them the right way, but it’s a bit complicated. Maybe for another question on SO(Stack Overflow) 😉
6. How do i get the day of week given a date?
Use weekday()
:
>>> import datetime
>>> datetime.datetime.today()
datetime.datetime(2012, 3, 23, 23, 24, 55, 173504)
>>> datetime.datetime.today().weekday()
4
From the documentation:
Return the day of the week as an integer, where Monday is 0 and Sunday is 6.
7. Convert pandas column to datetime?
Use the to_datetime
function, specifying a format to match your data.
raw_data['Mycol'] = pd.to_datetime(raw_data['Mycol'], format='%d%b%Y:%H:%M:%S.%f')
8. Get current time in milliseconds in python?
Using time.time()
:
import time
def current_milli_time():
return round(time.time() * 1000)
Then:
>>> current_milli_time()
1378761833768
9. How to change the datetime format in pandas?
You can use dt.strftime
if you need to convert datetime
to other formats (but note that then dtype
of column will be object
(string
)):
import pandas as pd
df = pd.DataFrame({'DOB': {0: '26/1/2016', 1: '26/1/2016'}})
print (df)
DOB
0 26/1/2016
1 26/1/2016
df['DOB'] = pd.to_datetime(df.DOB)
print (df)
DOB
0 2016-01-26
1 2016-01-26
df['DOB1'] = df['DOB'].dt.strftime('%m/%d/%Y')
print (df)
DOB DOB1
0 2016-01-26 01/26/2016
1 2016-01-26 01/26/2016
10. How to get current time in python and break up into year, month, day, hour, minute?
The datetime
module is your friend:
import datetime
now = datetime.datetime.now()
print(now.year, now.month, now.day, now.hour, now.minute, now.second)
# 2015 5 6 8 53 40
You don’t need separate variables, the attributes on the returned datetime
object have all you need.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |