When using a new library such as flask, you can improve you developer effectiveness by being familiar with the most common questions that come up when using the python flask library. Using the Stack Overflow Data Explorer tool, we’ve determined the top 10 most popular flask questions & answers by daily views on Stack Overflow to to be familiar with. Check out the top 10 flask questions & answers below:
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
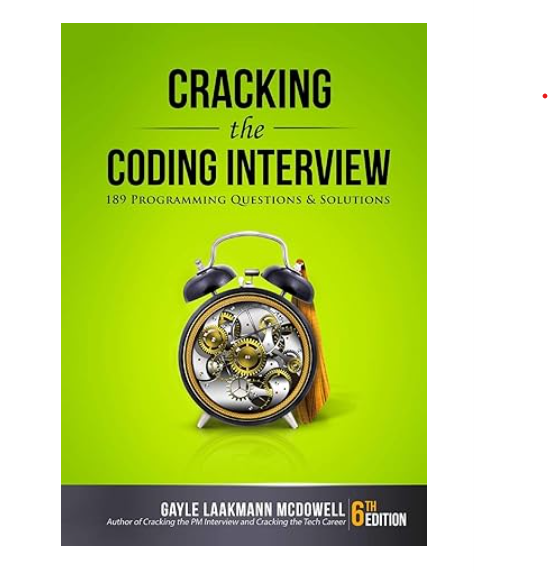
1. Why does my javascript code receive a “no ‘access-control-allow-origin’ header is present on the requested resource” error, while postman does not?
If I understood it right you are doing an XMLHttpRequest to a different domain than your page is on. So the browser is blocking it as it usually allows a request in the same origin for security reasons. You need to do something different when you want to do a cross-domain request. A tutorial about how to achieve that is Using CORS.
When you are using Postman they are not restricted by this policy. Quoted from Cross-Origin XMLHttpRequest:
Regular web pages can use the XMLHttpRequest object to send and receive data from remote servers, but they’re limited by the same origin policy. Extensions aren’t so limited. An extension can talk to remote servers outside of its origin, as long as it first requests cross-origin permissions.
2. Get the data received in a flask request?
The docs describe the attributes available on the request
object (from flask import request
) during a request. In most common cases request.data
will be empty because it’s used as a fallback:
request.data
Contains the incoming request data as string in case it came with a mimetype Flask does not handle.
request.args
: the key/value pairs in the URL query stringrequest.form
: the key/value pairs in the body, from a HTML post form, or JavaScript request that isn’t JSON encodedrequest.files
: the files in the body, which Flask keeps separate fromform
. HTML forms must useenctype=multipart/form-data
or files will not be uploaded.request.values
: combinedargs
andform
, preferringargs
if keys overlaprequest.json
: parsed JSON data. The request must have theapplication/json
content type, or userequest.get_json(force=True)
to ignore the content type.
All of these are MultiDict
instances (except for json
). You can access values using:
request.form['name']
: use indexing if you know the key existsrequest.form.get('name')
: useget
if the key might not existrequest.form.getlist('name')
: usegetlist
if the key is sent multiple times and you want a list of values.get
only returns the first value.
3. How to serve static files in flask?
The preferred method is to use NGINX or another web server to serve static files; they’ll be able to do it more efficiently than Flask.
However, you can use send_from_directory
to send files from a directory, which can be pretty convenient in some situations:
from flask import Flask, request, send_from_directory
# set the project root directory as the static folder, you can set others.
app = Flask(__name__, static_url_path='')
@app.route('/js/<path:path>')
def send_js(path):
return send_from_directory('js', path)
if __name__ == "__main__":
app.run()
Alternatively, you could use app.send_file
or app.send_static_file
, but this is highly discouraged as it can lead to security risks with user-supplied paths; send_from_directory
was designed to control those risks.
4. Flask importerror: no module named flask?
Try deleting the virtualenv you created. Then create a new virtualenv with:
virtualenv flask
Then:
cd flask
Now let’s activate the virtualenv
source bin/activate
Now you should see (flask)
on the left of the command line.
Edit: In windows there is no "source" that’s a linux thing, instead execute the activate.bat file, here I do it using Powershell: PS C:\DEV\aProject> & .\Flask\Scripts\activate
)
Let’s install flask:
pip install flask
Then create a file named hello.py
(NOTE: see UPDATE Flask 1.0.2
below):
from flask import Flask
app = Flask(__name__)
@app.route("/")
def hello():
return "Hello World!"
if __name__ == "__main__":
app.run()
and run it with:
python hello.py
UPDATE Flask 1.0.2
With the new flask release there is no need to run the app from your script. hello.py
should look like this now:
from flask import Flask
app = Flask(__name__)
@app.route("/")
def hello():
return "Hello World!"
and run it with:
FLASK_APP=hello.py flask run
Make sure to be inside the folder where hello.py
is when running the latest command.
All the steps before the creation of the hello.py apply for this case as well
5. How to get posted json in flask?
First of all, the .json
attribute is a property that delegates to the request.get_json()
method, which documents why you see None
here.
You need to set the request content type to application/json
for the .json
property and .get_json()
method (with no arguments) to work as either will produce None
otherwise. See the Flask Request
documentation:
This will contain the parsed JSON data if the mimetype indicates JSON (application/json, see
is_json()
), otherwise it will beNone
.
You can tell request.get_json()
to skip the content type requirement by passing it the force=True
keyword argument.
Note that if an exception is raised at this point (possibly resulting in a 400 Bad Request response), your JSON data is invalid. It is in some way malformed; you may want to check it with a JSON validator.
6. Return json response from flask view?
As of Flask 1.1.0 a view can directly return a Python dict and Flask will call jsonify
automatically.
@app.route("/summary")
def summary():
d = make_summary()
return d
If your Flask version is less than 1.1.0 or to return a different JSON-serializable object, import and use jsonify
.
from flask import jsonify
@app.route("/summary")
def summary():
d = make_summary()
return jsonify(d)
7. How can i get the named parameters from a url using flask?
Use request.args
to get parsed contents of query string:
from flask import request
@app.route(...)
def login():
username = request.args.get('username')
password = request.args.get('password')
8. How do i get flask to run on port 80?
1- Stop other applications that are using port 80.
2- run application with port 80 :
if __name__ == '__main__':
app.run(host='0.0.0.0', port=80)
9. How to use curl to send cookies?
This worked for me:
curl -v --cookie "USER_TOKEN=Yes" http://127.0.0.1:5000/
I could see the value in backend using
print(request.cookies)
10. Redirecting to url in flask?
You have to return a redirect:
import os
from flask import Flask,redirect
app = Flask(__name__)
@app.route('/')
def hello():
return redirect("http://www.example.com", code=302)
if __name__ == '__main__':
# Bind to PORT if defined, otherwise default to 5000.
port = int(os.environ.get('PORT', 5000))
app.run(host='0.0.0.0', port=port)
See the documentation on flask docs. The default value for code is 302 so code=302
can be omitted or replaced by other redirect code (one in 301, 302, 303, 305, and 307).
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |