To dynamically update your WordPress page’s Facebook Open Graph tags, first ensure you have the Yoast SEO plugin installed and enabled. We can dynamically update our Facebook Open Graph tags by intercepting the Yoast Facebook tag functions within the functions.php file found under the WordPress Dashboard Appearance->Editor.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
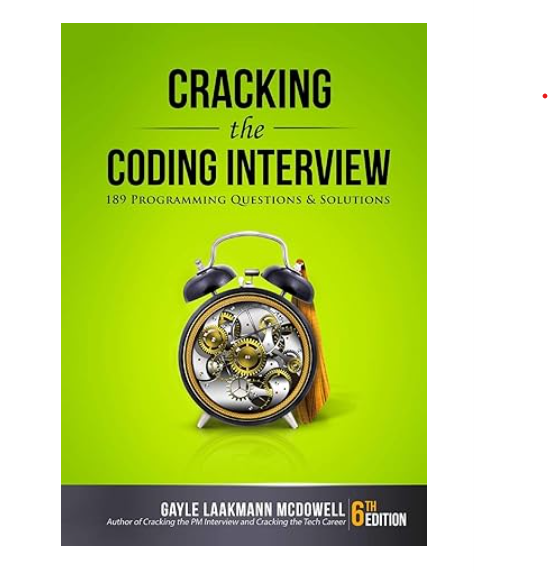
We use the add_filter
function to return our own open graph tag values when Yoast looks up the open graph values to populate in the page header:
add_action( 'wpseo_opengraph', 'change_yoast_seo_og_meta' ); function change_yoast_seo_og_meta() { /* Check if the post ID matches the post we want to update open graph tags for. */ if ( is_single ( 12345 ) ) { /* Add filters for existing yoast open graph tags. */ add_filter('wpseo_opengraph_desc', 'custom_opengraph_desc', 10, 1); add_filter('wpseo_opengraph_title', 'custom_opengraph_title'); add_filter('wpseo_opengraph_image', 'custom_opengraph_image', 10, 2); } } function custom_opengraph_desc( $desc ) { return "Updated open graph description!"; } function custom_opengraph_title( $title ) { return "Updated open graph title!"; } function custom_opengraph_image( $image ) { return "pericror.com/images/updatedImage.png"; }
In the example above, we update the open graph tag values for a given post with ID 12345. To get the post ID of a post, edit the post, and in the url of the page you will see the id after post=
. If the url was https://pericror.com/wp-admin/post.php?post=12345, the post ID would be 12345.
The example above shows you how to update the open graph tags, however they are still being set to static values! We can modify the custom functions to generate their return values dynamically. In our next example, we consider making a page that picks a random spirit animal when the page link is shared on Facebook. We generate the Facebook tags dynamically by setting our tags to a random set of description, title and image combinations:
add_action( 'wpseo_opengraph', 'change_yoast_seo_og_meta' ); function change_yoast_seo_og_meta() { /* Check if the post ID matches the post we want to update open graph tags for. */ if ( is_single ( 12345 ) ) { /* Define the new open graph tag data we will be using. */ global $spirit_animals; $spirit_animals = array( "owl", "lion", "fox"); global $animal_descriptions; $animal_descriptions = array( "The owl represents your wisdom.", "The lion represents your bravery.", "The fox represents your cunning."); global $animal_images; $animal_images = array( "https://pericror.com/wp-content/uploads/animals/owl.png", "https://pericror.com/wp-content/uploads/animals/lion.png", "https://pericror.com/wp-content/uploads/animals/fox.png"); global $random_animal; $random_animal = rand(0, count($spirit_animals)-1); /* Add filters for existing yoast open graph tags. */ add_filter('wpseo_opengraph_desc', 'spirit_animal_opengraph_desc', 10, 1); add_filter('wpseo_opengraph_title', 'spirit_animal_opengraph_title'); add_filter('wpseo_opengraph_image', 'spirit_animal_opengraph_image', 10, 2); } } function spirit_animal_opengraph_desc( $desc ) { return $GLOBALS['animal_descriptions'][$GLOBALS['random_animal']]; } function spirit_animal_opengraph_title( $title ) { $animal = $GLOBALS['spirit_animals'][$GLOBALS['random_animal']]; return "Your spirit animal is the " . $animal . "!"; } function spirit_animal_opengraph_image( $image ) { return $GLOBALS['animal_images'][$GLOBALS['random_animal']]; }
You should now have the tools to dynamically update your WordPress page’s Facebook open graph tags. One downside to dynamic Facebook open graph tags is that Facebook cache’s the open graph tags for a given page. You can force refresh the open graph tags manually using the open graph debugger, however a better solution is available. See the Programatically Force Update Facebook Open Graph post to learn how to use the Open Graph API and an Amazon EC2 free instance to keep the open graph tags updating.
The code used in this post is available on Github.
Was this blog helpful? Check out our top work from home essentials to stay comfortable and productive while you work:
HD Webcam | Noise Cancelling Headphones | HD Monitor | Ergonomic Keyboard and Mouse | Standing Desk |
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |