Under the WebRTC unified-plan sdp, it is preferred to utilize a single media stream with multiple video tracks when sending multiple videos across an RTC peer connection. In your js, you may call pc.addTransceiver('video', {direction: 'recvonly'});
multiple times to register multiple video tracks. In your pc.addEventListener(‘track’ track event listener callback, you may be calling document.getElementById(‘video’).srcObject = evt.streams[0]; but this defaults to the first track in the stream, even if multiple exist. So how do you select which video track to play from a WebRTC media stream in javascript?
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
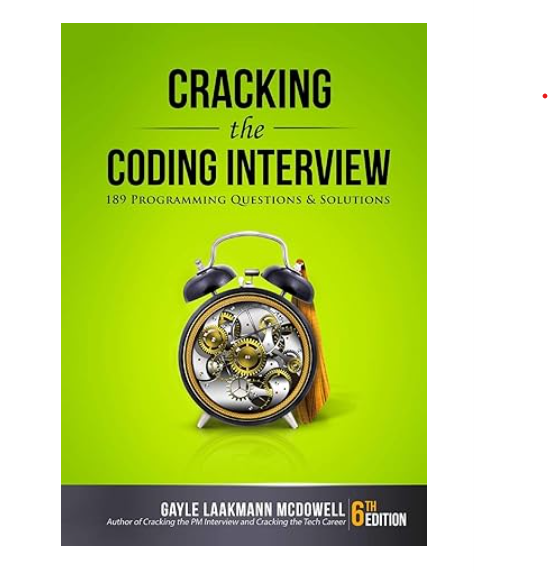
In the future, html5 video elements will support a videoTracks field whose selected
field will allow you to change which track to play (i.e videoElem.videoTracks[2].selected = true;
would play the third track in the media stream. Until this is supported, the workaround is to create an entirely new mediastream using the track you wish to play, and assigning it as the source of the video element:
pc.addEventListener('track', function(evt) { if (evt.track.kind == 'video') { video = document.createElement('video'); video.srcObject = new MediaStream([evt.track]); document.getElementById('video-container').appendChild(video) } });
Above, we use the RTCPeerConnection track event to convert the track into a new mediastream and assign it to the video source. This allows us assign a newly received track from a media stream to the video stream, even if the track is not the first track in the media stream. Alternatively we can access the media stream from the track event and choose which track to assign, i.e the third track (index 2) below:
video.srcObject = new MediaStream([evt.streams[0].tracks[2]]);
We hope with this tutorial you learned how to select a video track to play from a WebRTC media stream in javascript.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |